To access the Butler API, you'll need an API Key.
How to get your API Key
You can find your API Key in the Settings > API Key section of the Butler app:
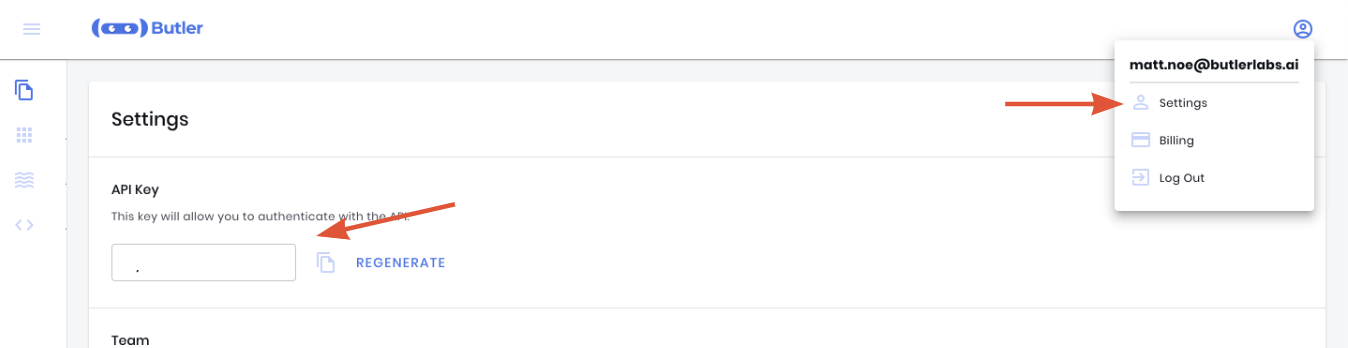
If this is your first time using the API, you'll need to generate a new API Key by clicking on the Regenerate button
Never give your API Key to a third party
Your API Key can give access to your private Butler data and should be treated like a password. Make sure to keep it secure!
How to use your API Key
Once you have your API Key, simply provide it as an Authorization:Bearer
header in your requests, as shown below. If you want to more deeply understand how the Authorization:Bearer
header works, feel free to check out the full spec here .
curl --request GET \
--url https://app.butlerlabs.ai/api/queues/{queueId}/extraction_results \
--header 'Accept: application/json' \
--header 'Authorization: Bearer <api_key>'
import requests
url = "https://app.butlerlabs.ai/api/queues/{queueId}/extraction_results"
headers = {
"Accept": "application/json",
"Authorization": "Bearer <api_key>"
}
response = requests.request("GET", url, headers=headers)
const fetch = require('node-fetch');
const url = 'https://app.butlerlabs.ai/api/queues/{queueId}/extraction_results';
const options = {
method: 'GET',
headers: {
Accept: 'application/json',
Authorization: 'Bearer <api_key>'
}
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));