Overview
This guide will help you extract data from Driver's License using Butler's OCR APIs in Python. In 15 minutes you'll be ready to add Python Driver's License OCR into your product or workflow!
Before getting started, you'll want to make sure to do the following:
- Signup for a free Butler account at https://app.butlerlabs.ai
- Write down your Butler API key from the Settings menu. Follow the Getting Started guide for more details about how to do that.
Get your API ID
Sign into the Butler product, go to the Library and search for the US Driver's License model:
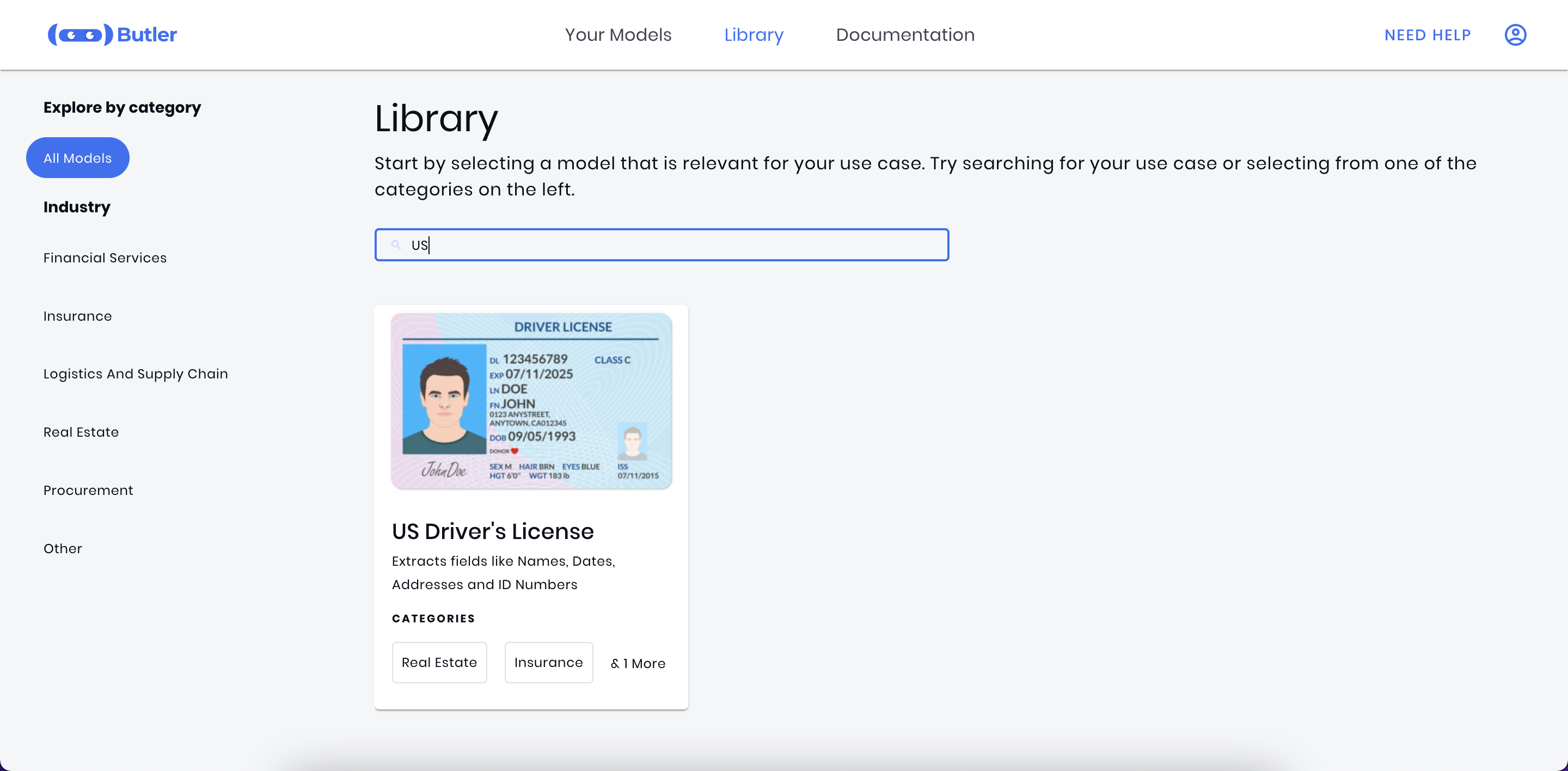
Click on the US Driver's License card, then press the Create button to create a new US Driver's License model:
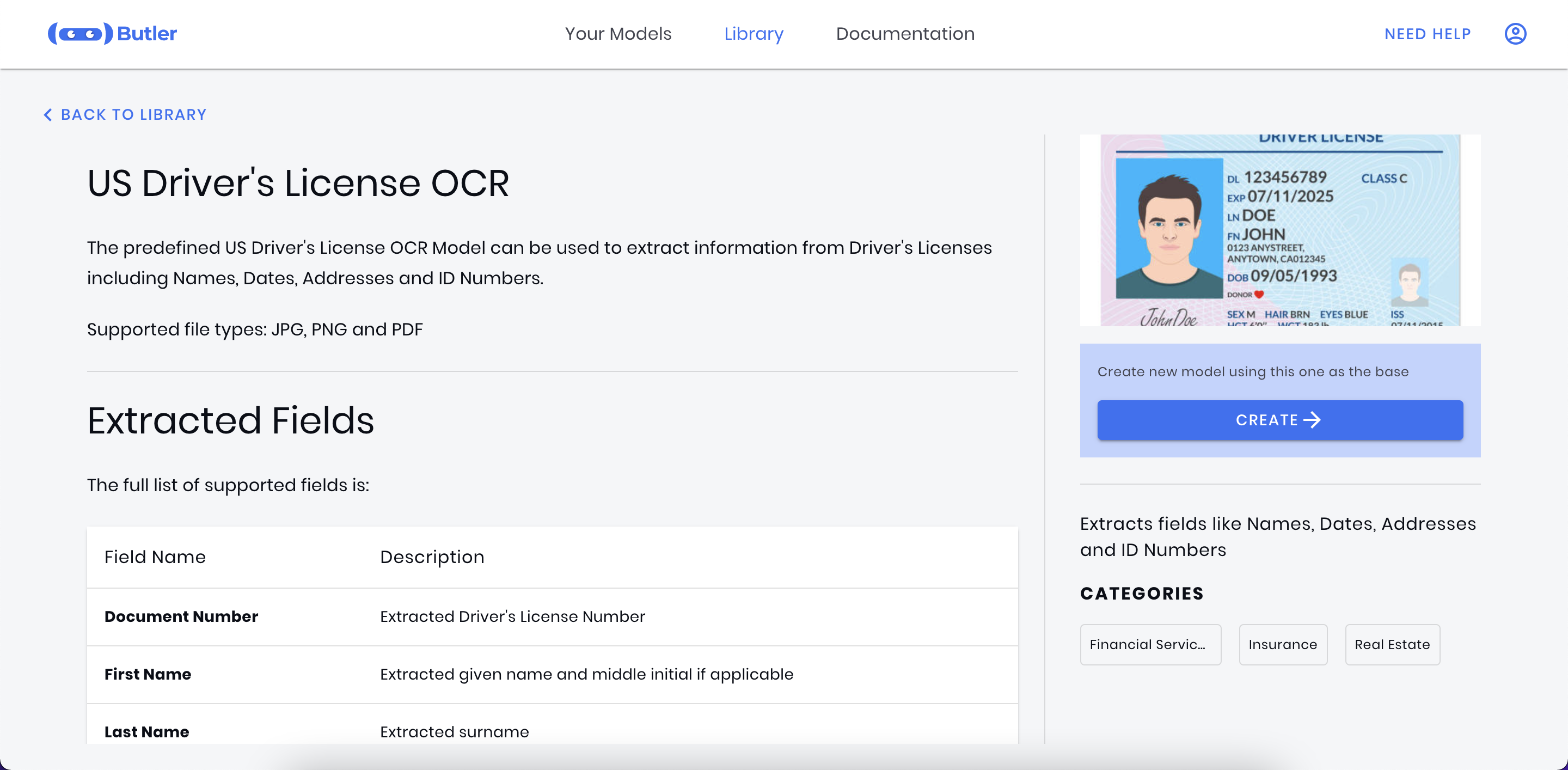
Once on the model details page, go to the APIs tab:
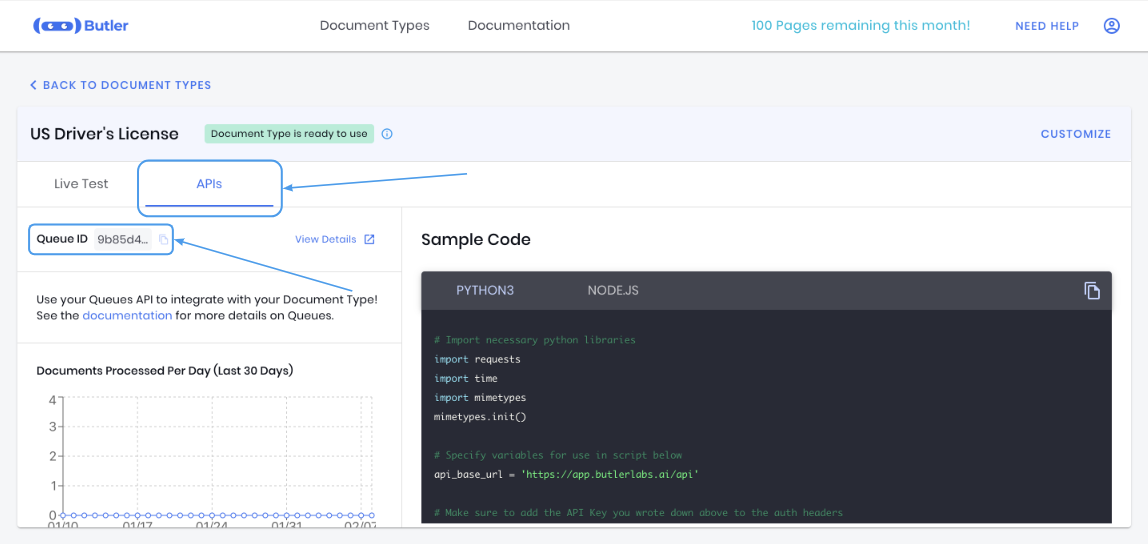
Copy the API ID (also known as the Queue ID) and write it down. We'll use it in our code below.
Sample Python Driver's License OCR Code
You can copy and paste the following Python sample code to process documents with OCR using the API.
# Ensure it's installed in your environment with pip install butler-sdk
from butler import Client
# Get API Key from https://docs.butlerlabs.ai/reference/uploading-documents-to-the-rest-api#get-your-api-key
api_key = '<api-key>'
# Get Queue ID from https://docs.butlerlabs.ai/reference/uploading-documents-to-the-rest-api#go-to-the-model-details-page
queue_id = '<queue_id>'
# Response is a strongly typed object
response = Client(api_key).extract_document(queue_id, 'sample_dl.jpeg')
# Convert to a dictionary for printing
print(response.to_dict())
In-Product Sample Code
You can also copy the sample code directly from the product. This code will have your API ID and API Key already pre-populated for you!
Extracted Driver's License Fields
Here is an example of what a Driver's License JSON response looks like:
{
"documentId": "1540d070-c500-4994-a0bc-4196499c41de",
"documentStatus": "Completed",
"fileName": "alabama.jpeg",
"mimeType": "image/jpeg",
"documentType": "US Driver's License",
"confidenceScore": "High",
"formFields": [
{
"fieldName": "Document Number",
"value": "1234567",
"confidenceScore": "High"
},
{
"fieldName": "First Name",
"value": "CONNOR",
"confidenceScore": "Low"
},
{
"fieldName": "Last Name",
"value": "SAMPLE",
"confidenceScore": "High"
},
{
"fieldName": "Birth Date",
"value": "01-05-1948",
"confidenceScore": "High"
},
{
"fieldName": "Expiration Date",
"value": "01-05-2014",
"confidenceScore": "High"
},
{
"fieldName": "Address",
"value": "1 WONDERFUL DRIVE MONTGOMERY AL 36104-1234",
"confidenceScore": "High"
},
{
"fieldName": "Sex",
"value": "SEXM",
"confidenceScore": "High"
},
{
"fieldName": "State",
"value": "Alabama",
"confidenceScore": "High"
}
],
"tables": []
}
Driver's License API Response Details
For more details on each of the fields returned from the Driver's License API, see the Driver's License page.